Hey there, fellow coder! If you've ever wondered how to create a struct in C, you've come to the right place. Structs are like the building blocks of your code—they help organize data in a way that makes sense. Think of them as a custom data type that lets you group related variables together. Whether you're working on a simple project or building something more complex, understanding structs is essential. So, buckle up and let's dive into the world of C programming!
Before we get too deep into the nitty-gritty, let's talk about why you should care about structs. In C, you can define your own data types using structs, which is super handy when you're dealing with multiple variables that need to work together. Imagine you're building a program to manage a library. Instead of having separate arrays for book titles, authors, and genres, you can create a single struct called "Book" that holds all this info. Sounds neat, right?
Now, don't worry if you're new to this. We'll break it down step by step, from the basics of what a struct is to advanced tips and tricks. By the end of this guide, you'll be a struct-savvy coder ready to tackle any project. So, grab your favorite coding snack and let's get started!
Read also:Tuesday Dad Jokes The Ultimate Collection To Brighten Your Week
What is a Struct in C?
Alright, let's start with the basics. A struct in C is essentially a user-defined data type that allows you to combine different types of data under one roof. Think of it like a container where you can store related variables. For example, if you're building a program for a school, you might have a struct called "Student" that holds the student's name, ID, grade, and attendance record. This makes your code cleaner, more organized, and easier to manage.
Here's the cool part: structs can hold variables of different data types, such as integers, floats, characters, and even other structs. This flexibility is what makes them so powerful. Plus, once you define a struct, you can create as many instances (or "objects") of it as you need. It's like having a blueprint for creating data structures tailored to your specific needs.
Now, let me give you a quick example of what a struct looks like in C:
struct Student {
char name[50];
int id;
float gpa;
};
See how we grouped the student's name, ID, and GPA into a single struct? This is just the beginning. Let's move on to the next section to see how you can actually create and use structs in your code.
How to Create a Struct in C
Creating a struct in C is pretty straightforward. All you need to do is use the "struct" keyword followed by the name of your struct and a list of member variables inside curly braces. Here's the basic syntax:
Read also:Taylor Rs Husband The Untold Story And Everything You Need To Know
struct [struct_name] {
[data_type] [member_name1];
[data_type] [member_name2];
...
};
Let's break this down. The "struct" keyword tells the compiler that you're defining a new data type. The [struct_name] is the name you give to your struct, and the member variables are the individual pieces of data you want to store inside it. You can have as many member variables as you need, and they can be of any valid C data type.
For example, if you want to create a struct called "Car" that holds information about a car's make, model, and year, you could write:
struct Car {
char make[20];
char model[20];
int year;
};
Now that you know how to define a struct, let's talk about how to use it in your code. We'll cover that in the next section.
Using Structs in Your Code
Once you've defined a struct, you can create instances of it and access its members using the dot operator (.). Here's how it works:
struct Car myCar;
strcpy(myCar.make, "Toyota");
strcpy(myCar.model, "Corolla");
myCar.year = 2022;
In this example, we created a variable called "myCar" of type "Car" and assigned values to its member variables. The strcpy function is used to copy strings into the "make" and "model" fields, while the "year" field is assigned directly since it's an integer.
One thing to note: when you create a struct variable, it doesn't automatically initialize its members. You'll need to assign values to them yourself. This gives you more control over your data, but it also means you need to be careful to avoid using uninitialized variables.
Accessing Struct Members
Accessing struct members is super easy. Just use the dot operator followed by the member name. For example:
printf("Make: %s\n", myCar.make);
printf("Model: %s\n", myCar.model);
printf("Year: %d\n", myCar.year);
This will print out the values of the "make," "model," and "year" members of the "myCar" struct. Simple, right?
Why Use Structs in C?
Now that you know how to create and use structs, you might be wondering why they're so useful. Here are a few reasons:
- Organization: Structs help you organize related data into a single unit, making your code easier to read and maintain.
- Reusability: Once you define a struct, you can create as many instances of it as you need, reducing code duplication.
- Flexibility: Structs can hold variables of different data types, giving you the freedom to design data structures that fit your specific needs.
- Portability: Structs can be passed to functions as arguments or returned as values, making them a versatile tool in your coding arsenal.
These are just a few of the many benefits of using structs in C. As you gain more experience, you'll discover even more ways to leverage their power.
Advanced Struct Features
Now that you've got the basics down, let's explore some advanced features of structs in C. These will take your struct skills to the next level:
Nested Structs
You can define a struct inside another struct, which is known as a nested struct. This is useful when you have complex data structures that need to be broken down into smaller parts. For example:
struct Address {
char street[50];
char city[30];
char state[20];
};
struct Person {
char name[50];
int age;
struct Address addr;
};
In this example, the "Person" struct contains an "Address" struct as one of its members. This allows you to group related data together in a logical way.
Pointers to Structs
Sometimes, you might want to work with pointers to structs instead of the structs themselves. This can be more efficient, especially when dealing with large data structures. Here's how it works:
struct Person *personPtr;
personPtr = malloc(sizeof(struct Person));
strcpy(personPtr->name, "John Doe");
personPtr->age = 30;
Notice the arrow operator (->) used to access struct members through a pointer. This is a common pattern in C programming and can make your code more efficient and flexible.
Structs and Functions
Structs can be passed to and returned from functions, which makes them incredibly versatile. Here's an example:
struct Car getCarDetails() {
struct Car car;
strcpy(car.make, "Honda");
strcpy(car.model, "Civic");
car.year = 2021;
return car;
}
In this example, the "getCarDetails" function returns a struct of type "Car." You can then use this struct in your main program or pass it to other functions as needed.
Common Mistakes to Avoid
When working with structs in C, there are a few common mistakes that beginners often make. Here are some tips to help you avoid them:
- Forgetting to Initialize Members: Always make sure to initialize the members of your structs before using them.
- Using Incorrect Data Types: Double-check that the data types of your struct members match the data you're trying to store.
- Accessing Out-of-Bounds Memory: Be careful when working with arrays inside structs to avoid accessing memory that doesn't belong to your program.
- Forgetting to Free Memory: If you're using dynamic memory allocation with structs, remember to free the memory when you're done with it.
By keeping these tips in mind, you'll be able to write cleaner, more reliable code.
Real-World Applications of Structs
Structs are used in a wide variety of real-world applications. Here are a few examples:
- Database Systems: Structs are often used to represent rows in a database, where each member corresponds to a column.
- Graphics Programming: Structs can be used to represent points, vectors, and other geometric objects in graphics applications.
- Network Protocols: Structs are commonly used to define the format of data packets in network communications.
- Game Development: Structs can be used to represent game objects, such as characters, weapons, and items.
These are just a few examples of how structs are used in practice. As you gain more experience, you'll find even more ways to apply them to your projects.
Conclusion
Well, there you have it—everything you need to know about creating a struct in C. Structs are a powerful tool that can help you organize your data, write cleaner code, and solve complex problems. Whether you're a beginner or an experienced coder, mastering structs is an essential skill in the world of C programming.
So, what are you waiting for? Start experimenting with structs in your own projects and see how they can transform the way you code. And don't forget to share your experiences and ask questions in the comments below. Happy coding, and see you in the next article!
Table of Contents
- What is a Struct in C?
- How to Create a Struct in C
- Using Structs in Your Code
- Why Use Structs in C?
- Advanced Struct Features
- Structs and Functions
- Common Mistakes to Avoid
- Real-World Applications of Structs
- Conclusion


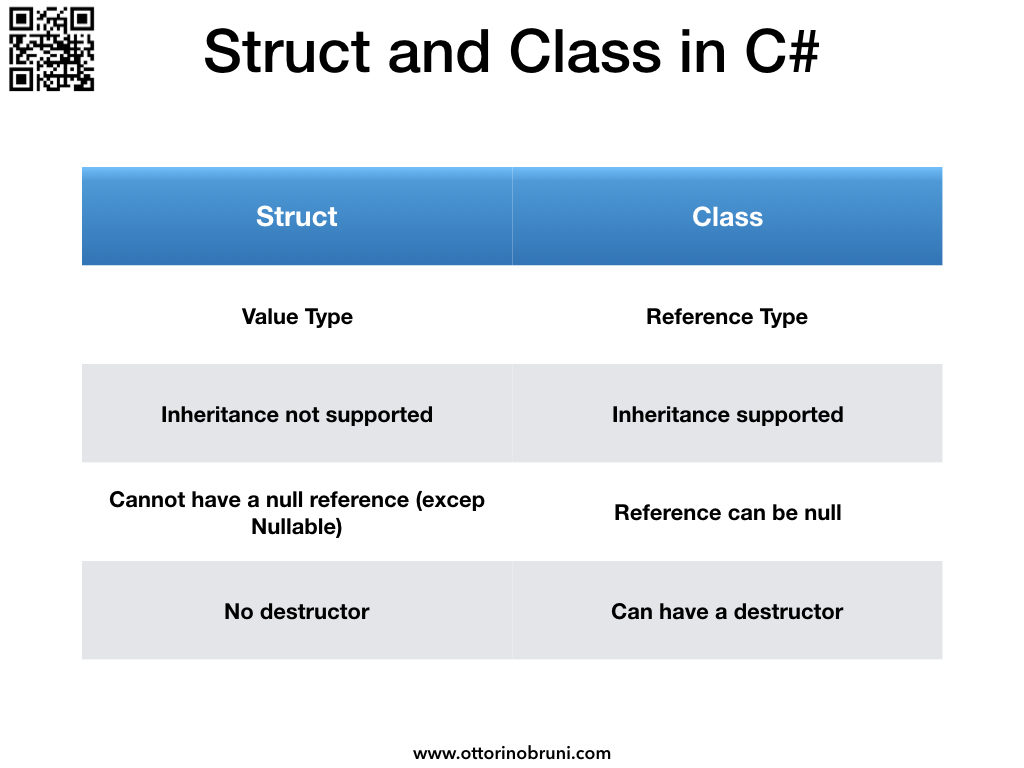